COVID19 Lockdown Dev Log – Day 24, Debugging In NodeJs
What I Worked On
Finishing the Notes App in NodeJs
What I Learned
By using “debugger” in your code and “node inspect” in the terminal you can debug your script by pausing it in certain places. This is handy for checking current values of for example variables, functions etc.
Let’s look at an example by using my Notes App. It’s an app for writing notes using the terminal/commandline. In this app I have a function called ‘addNote’. This function also uses another function to load the file with other notes, which is stored in the const ‘notes’. I won’t show the code for it, just know that it fetches the notes from another file:
const addNote = (title, body) => {
const notes = loadNotes()
const duplicateNote = notes.find(( note ) => note.title === title)
if (!duplicateNote) {
notes.push({
title: title,
body: body
})
saveNotes(notes)
console.log(chalk.black.bgGreen('New note added'))
} else {
console.log(chalk.white.bgRed('Note title already taken'))
}
}
Let’s say that we want to find out if the ‘notes’ are fetched before the ‘addNote’ function continues to push a new note to the list of notes. We add a “debugger” in our code:
const addNote = (title, body) => {
const notes = loadNotes()
const duplicateNote = notes.find(( note ) => note.title === title)
debugger
//... the rest of the code for addNote
}
Then we use the “inspect” command, provided by NodeJs, in the terminal to run the script, which in this case is ‘app.js’:
node inspect app.js add --title="Debugging NodeJs" --body="this is how u debug in nodejs"
Note: The ‘add’ command is a custom command created in the ‘app.js’ script
The terminal/commandline enters the debug state and gives us the following output:
< Debugger listening on ws://127.0.0.1:9229/aa178b09-d11b-4b64-9802-68e400f3797e
< For help, see: https://nodejs.org/en/docs/inspector
< Debugger attached.
Break on start in app.js:1
> 1 const yargs = require('yargs');
2 const notes = require('./note.js');
3
debug>
Next, we open Chrome and enter the URL “chrome://inspect”, which takes us to a page listing the targets that we can debug. We have only one target, which is our Notes App:
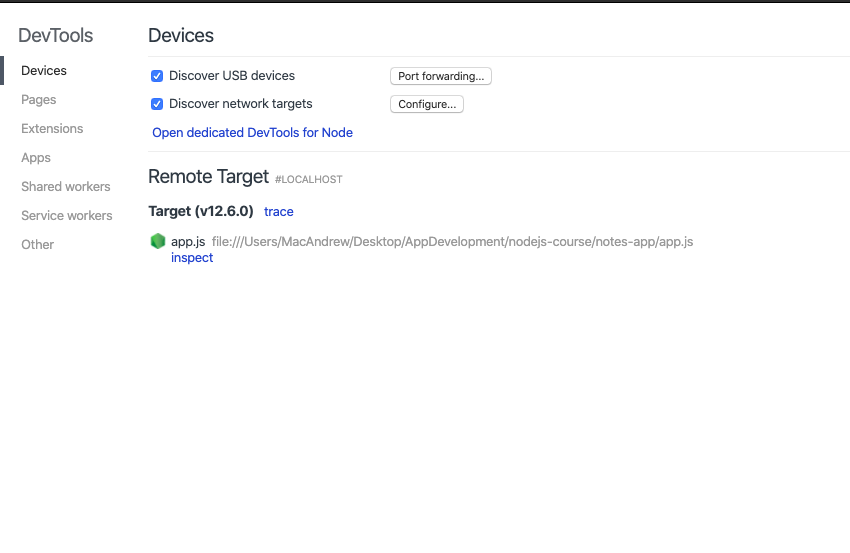
When we click ‘inspect’ we are presented with a Developer Tools window:
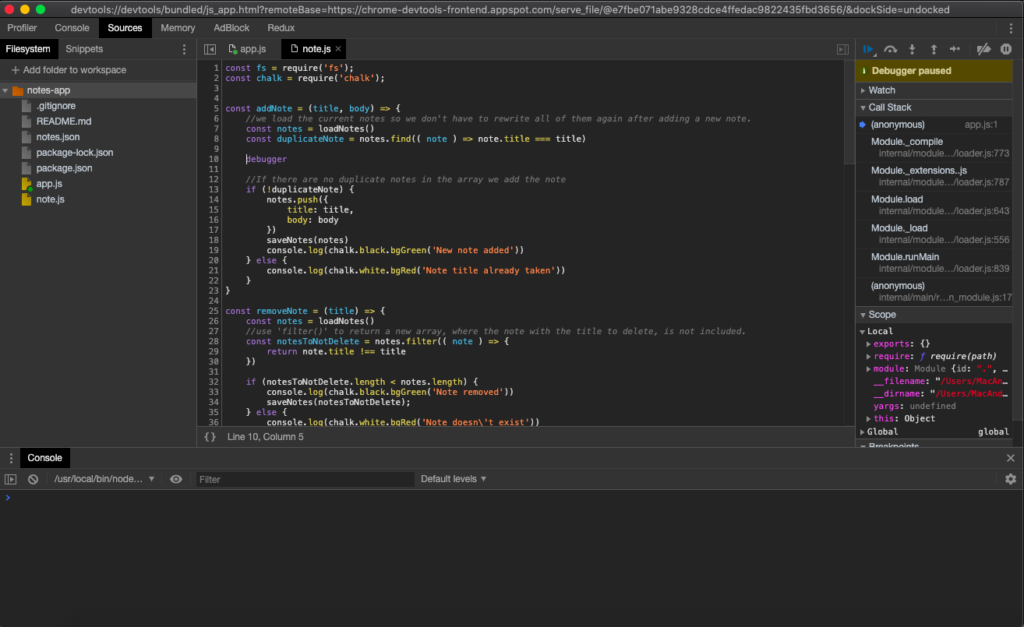
To the left we have our workspace (if it’s empty, you can add it by clicking “Add folder to workspace). I won’t go into depth with the whole Developer Tools window here, so to keep it simple, we see the ‘app.js’ tab and ‘notes.js’ tab, where we have the ‘notes.js” tab open. This is where the ‘addNote’ function is. For now, the script is paused, but we can run it by clicking on the blue pause/run icon the top right corner. The script will execute until it hits the “debugger” in our code. This way we can now see what value our ‘const notes’ has:
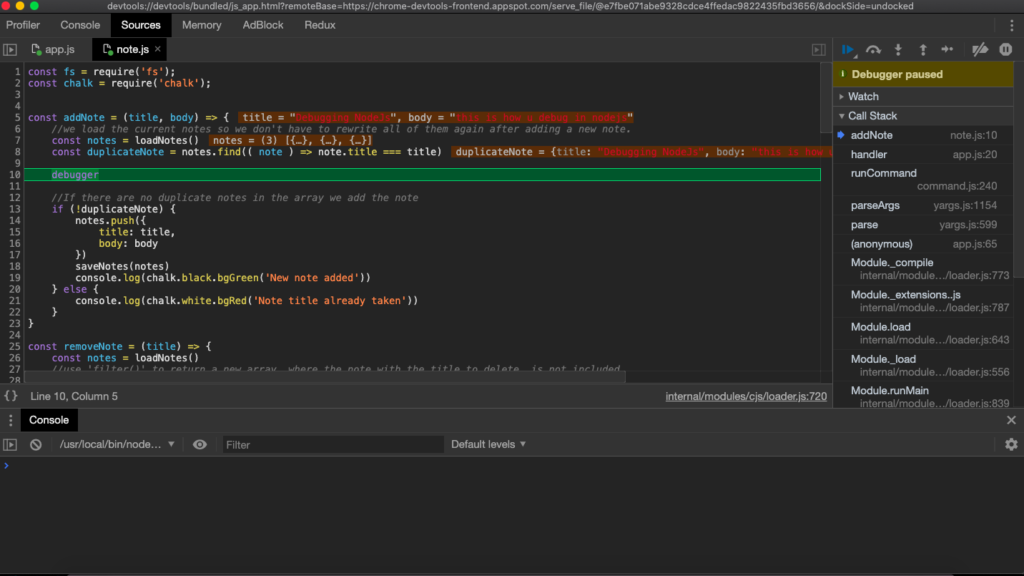
And there we have it! 😀 You can resume the execution of the script by pressing run/pause again, which in this case will run it till the end. If I want to restart it, I can type ‘restart’ in my terminal/command 😀
What Distracted Me During The Day
- GF needed help with translating an email from Danish to English
Resources
- NodeJs debugging – https://nodejs.org/en/docs/guides/debugging-getting-started/