COVID19 Lockdown Dev Log – Day 21, String.prototype.replace with Regex
What I Worked On
Using regex (regular expression) to split string data into new lines.
What I Learned
Note: This is a follow-up on yesterday’s post 😀
I learned to make better use of the online tool 101regex by using the ‘substitution’ window. It basically shows how your regex will output your pattern with replacement values, which helped me visualize my regex pattern. You can see how I made my regex with the tool here. In JavaScript I applied the pattern with the String.prototype.replace (or just ‘replacet()’) function again. So instead of a nested foreach loop like this:
// index.js
//...code
//first foreach loop
lists.each((index, element) => {
const articles = []
const title = $(element).find('.list__title').text();
const list = $(element).find('.list-article__title')
if(title === "Seneste plus") {
return
}
//nested foreach loop
list.each((i, item) => {
const listItem = $(item).text();
articles.push(`${listItem}\n`)
});
//Write to CSV
writeStream.write(`${title} \n ${articles} \n`);
})
I managed to rewrite it with the regex like this:
// index.js
//...code
lists.each((index, element) => {
const title = $(element).find('.list__title').text();
const list = $(element)
.find('.list-article__title')
.text()
.replace(/([a-z])([A-Z])/g, "$1 \n$2");
if(title === "Seneste plus") {
return
}
//Write to CSV
writeStream.write(`${title} \n ${list} \n`);
})
It looks a bit cleaner in my opinion 😀 The output looks like this in a terminal:
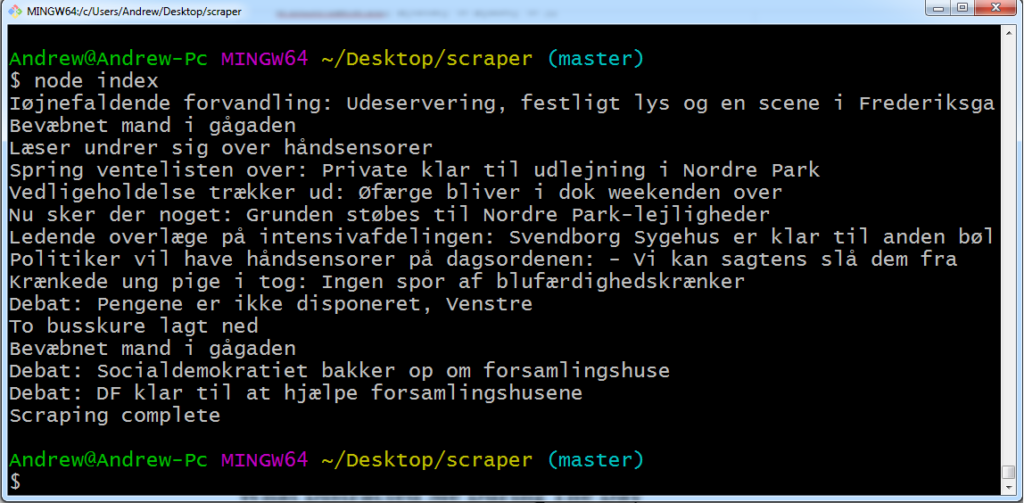
And Like this in MS Excel:
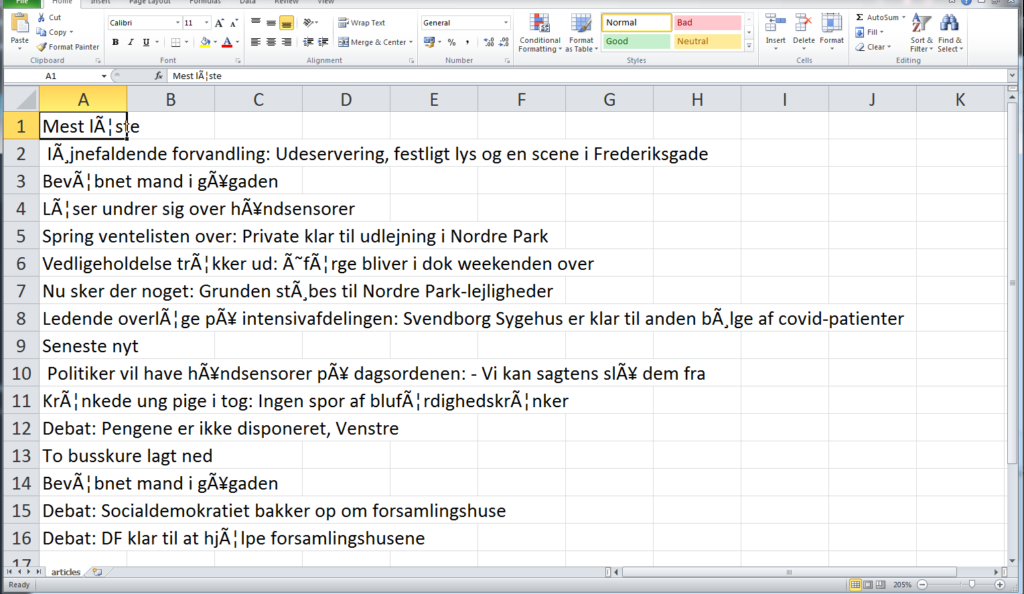
I need to learn how to write scraped data to a ‘.CSV’ file in a better way, but I’ll take this for now 😀 I got to experiment and learn more about regex. Maybe I should look into building a frontend for the scraper and put it live 😀
What Distracted Me During The Day
- Emails